Java SE 21 Developer (1Z0-830) by Simon Roberts
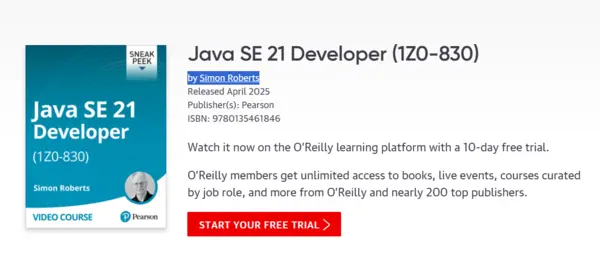
Free Download Java SE 21 Developer (1Z0-830) by Simon Roberts
Released 4/2025
By Simon Roberts
MP4 | Video: h264, 1280x720 | Audio: AAC, 44.1 KHz, 2 Ch
Level: Beginner | Genre: eLearning | Language: English + subtitle | Duration: 20h 12m | Size: 4.44 GB
Table of contents
Module 1: Working with Java Data Types
Module Introduction
Lesson 1: Operations and core data types
Learning objectives
1.1 Java operators–Part 1
1.2 Java operators–Part 2
1.3 Promotions
1.4 Casting
1.5 Wrapper classes
1.6 Primitives, references, aliasing, and equality
1.7 Question deep dive
Lesson 2: Handling text
Learning objectives
2.1 String and StringBuilder
2.2 Methods of the String class
2.3 Text blocks
2.4 Methods of the StringBuilder class
2.5 Question deep dive
Lesson 3: The date-time API
Learning objectives
3.1 Date-time API foundations–Part 1
3.2 Date-time API foundations–Part 2
3.3 Manipulating date-time objects
3.4 Time-zone considerations–Part 1
3.5 Time-zone considerations–Part 2
3.6 Time-zone considerations–Part 3
3.7 Question deep dive
Module 2: Controlling Program Flow and Exception Handling
Module Introduction
Lesson 4: Fundamentals of flow control
Learning objectives
4.1 Simple loops
4.2 Control using break and continue
4.3 Using if/else statements
4.4 Using switch statements
4.5 The arrow form of switch
4.6 Expressions with switch
4.7 Pattern matching with switch
4.8 Question deep dive
Lesson 5: Flow control with exceptions
Learning objectives
5.1 Flow control with try/catch/finally
5.2 Flow control with try-with-resources
5.3 Multi-catch and rethrowing
5.4 Implementing AutoCloseable–Part 1
5.5 Implementing AutoCloseable–Part 2
5.6 Question deep dive
Lesson 6: Custom exceptions
Learning objectives
6.1 Subclassing Throwable types
6.2 Question deep dive
Module 3: Java Object-Oriented Approach
Module Introduction
Lesson 7: Class definition, and reachability
Learning objectives
7.1 Source files and basic type declarations
7.2 Nested type declarations
7.3 Inner class declarations–Part 1
7.4 Inner class declaration–Part 2
7.5 Local and anonymous class declarations
7.6 Reachability analysis
7.7 Question deep dive
Lesson 8: Defining class contents
Learning objectives
8.1 Instance and static fields–Part 1
8.2 Instance and static fields–Part 2
8.3 Instance and static methods–Part 1
8.4 Instance and static methods–Part 2
8.5 Variable length argument handling
8.6 Overloaded and overridden methods–Part 1
8.7 Overloaded and overridden methods–Part 2
8.8 Defining records
8.9 Features of records
8.10 Question deep dive
Lesson 9: Initialization of objects
Learning objectives
9.1 Static initialization
9.2 Instance initialization
9.3 Question deep dive
Lesson 10: Scope, encapsulation, and immutability
Learning objectives
10.1 Rules of scope
10.2 Access control modifiers
10.3 Encapsulation
Requirements
10.4 Immutability
Requirements
10.5 Question deep dive
Lesson 11: Local variable type inference
Learning objectives
11.1 Using var for regular variables
11.2 Additional uses and restrictions of var
11.3 Question deep dive
Lesson 12: Implementation inheritance
Learning objectives
12.1 Subclass declaration
12.2 Subclass initialization
12.3 Abstract class constraints
12.4 Sealed type hierarchies
12.5 Special cases in sealed type hierarchies
12.6 Question deep dive
Lesson 13: Working with polymorphism
Learning objectives
13.1 Object and reference type
13.2 Pattern matching in instanceof
13.3 Additional topics in pattern-matched instanceof
13.4 Possible and impossible casts
13.5 Virtual method invocation
13.6 Covariant returns
13.7 Question deep dive
Lesson 14: Interfaces
Learning objectives
14.1 Interfaces, methods and functional interfaces
14.2 Interface implementation
14.3 Default method resolution
14.4 Question deep dive
Lesson 15: Enumerations
Learning objectives
15.1 Enum values and initialization
15.2 Enum fields and methods
15.3 Question deep dive
Module 4: Working with Arrays and Collections
Module Introduction
Lesson 16: Generics and generic declarations
Learning objectives
16.1 Fundamentals of generics
16.2 Declaring generic types and methods
16.3 Using bounds and wildcards
16.4 Question deep dive
Lesson 17: Java core collections
Learning objectives
17.1 Arrays, and methods of Collection, List, and Set–Part 1
17.2 Arrays, and methods of Collection, List, and Set–Part 2
17.3 Methods of Deque and Map
17.4 Question deep dive
Lesson 18: Comparator, Comparable, and ordering
Learning objectives
18.1 Comparison methods and interfaces
18.2 Sorting arrays and Collections
18.3 Comparator factories and decorators
18.4 Question deep dive
Module 5: Working with Streams and Lambda Expressions
Module Introduction
Lesson 19: Defining lambda expressions
Learning objectives
19.1 Lambda expression syntax variations
19.2 Lambda expression contexts
19.3 Core functional interfaces
19.4 Method references
19.5 Question deep dive
Lesson 20: Fundamental Stream operations
Learning objectives
20.1 The monad-like methods
20.2 Stream utilities
20.3 Simple terminal methods and laziness
20.4 Question deep dive
Lesson 21: Reduction operations, and parallelism
Learning objectives
21.1 Collection and reduction–Part 1
21.2 Collection and reduction–Part 2
21.3 Grouping and partitioning with Collectors
21.4 Downstream operations with Collectors
21.5 Parallel stream operation
21.6 Question deep dive
Module 6: Java Platform Module System
Module Introduction
Lesson 22: Building and executing modules
Learning objectives
22.1 Module compilation
22.2 Module execution
22.3 Question Deep Dive
Lesson 23: Coding modules
Learning objectives
23.1 Exports and requires directives
23.2 Provides, uses, open and opens directives
23.3 Question Deep Dive
Lesson 24: Migration, and command line operations
Learning objectives
24.1 Project Migration–Part 1
24.2 Project Migration–Part 2
24.3 Command-line utilities
24.4 Question Deep Dive
Module 7: Concurrency
Module Introduction
Lesson 25: Create and execute threads
Learning objectives
25.1 Runnable and Thread
25.2 ExecutorService and Future
25.3 ExecutorService lifecycle–Part 1
25.4 ExecutorService lifecycle–Part 2
25.5 Virtual threads 12 m
25.6 Question Deep Dive
Lesson 26: Thread-safe code, locking, and synchronization
Learning objectives
26.1 Race conditions, deadlock, and livelock
26.2 Transactional integrity
26.3 Visibility
26.4 Concurrent queues and collections
26.5 Synchronizers, locks, and atomic types–Part 1
26.6 Synchronizers, locks, and atomic types–Part 2
26.7 Question Deep Dive
Module 8: Java IO
Module Introduction
Lesson 27: Fundamental IO operations
Learning objectives
27.1 Input and Output streams, Reader and Writer
27.2 BufferedReader, PrintWriter, Scanner and Charset conversions
27.3 Question Deep Dive
Lesson 28: Java serialization
Learning objectives
28.1 Default serialization
28.2 Customizing serialization
28.3 Question Deep Dive
Lesson 29: Files, Path, and Channel
Learning objectives
29.1 Files methods–Part 1
29.2 Files methods–Part 2
29.3 Working with Channel
29.4 Question Deep Dive
Module 9: Localization in Java SE Applications
Module Introduction
Lesson 30: Localization
Learning objectives
30.1 Parsing, formatting, and locale
30.2 ResourceBundle and data lookup
30.3 Question Deep Dive
Summary
Java SE 21 Developer (1Z0-830): Summary
https://www.oreilly.com/library/view/java-se-21/9780135461846/
Buy Premium From My Links To Get Resumable Support,Max Speed & Support Me
AusFile
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part1.rar.html
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part2.rar.html
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part3.rar.html
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part4.rar.html
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part5.rar.html
Fileaxa
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part1.rar
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part2.rar
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part3.rar
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part4.rar
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part5.rar
TakeFile
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part1.rar.html
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part2.rar.html
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part3.rar.html
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part4.rar.html
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part5.rar.html
Rapidgator
PearsonJavaSE21Developer1Z0830.html
http://peeplink.in/bdd9f9df76c0
Fikper
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part1.rar.html
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part2.rar.html
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part3.rar.html
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part4.rar.html
vpsob.Pearson..Java.SE.21.Developer.1Z0830.part5.rar.html
No Password - Links are Interchangeable
Free Download Java SE 21 Developer (1Z0-830) by Simon Roberts is known for its high-speed downloads. It uses multiple file hosting services such as Rapidgator.net, Nitroflare.com, Uploadgig.com, and Mediafire.com to host its files